Maybe this will help?
// Line drawing function
DrawContext.prototype.drawLine = function (p_x1, p_y1, p_x2, p_y2, p_colourLine, p_intWidth)
{
let pthLine = new Path();
pthLine.move(new Point(p_x1, p_y1));
pthLine.addLine(new Point(p_x2, p_y2));
this.addPath(pthLine);
this.setStrokeColor(p_colourLine);
this.setLineWidth(p_intWidth);
this.strokePath();
}
// Set-up a small widget and drawing region
let wgtMain = new ListWidget();
wgtMain.setPadding(0, 0, 0, 0);
wgtMain.backgroundColor = new Color("#F0F9F4")
let dcRegion = new DrawContext();
dcRegion.size = new Size(282, 282);
dcRegion.opaque = true;
// Draw some lines
dcRegion.drawLine(20, 20, 180, 180, Color.red(), 10);
dcRegion.drawLine(20, 20, 20, 250, Color.green(), 8);
dcRegion.drawLine(20, 20, 150, 20, Color.blue(), 3);
dcRegion.drawLine(100, 100, 100, 200, Color.purple(), 5);
dcRegion.drawLine(100, 100, 200, 100, Color.gray(), 5);
// Render the widget with the lines on it
wgtMain.backgroundImage = dcRegion.getImage();
if (!config.runsInWidget) await wgtMain.presentSmall();
Script.setWidget(wgtMain);
Script.complete();
It should produce the following on a small widget.
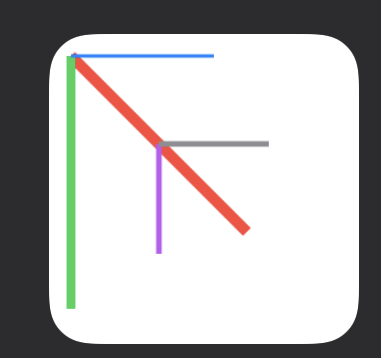
But, do make sure to have a read through the Scriptable documentation at the functions and look through the examples for widgets you can find posted on the forum. From those you should be able to develop an understanding of how everything fits together. Experiment with them and it should help you get the sort of level of understanding I think you may be looking for,
Hope that helps.